Javascript Pro: Mastering Advanced Concepts And Techniques
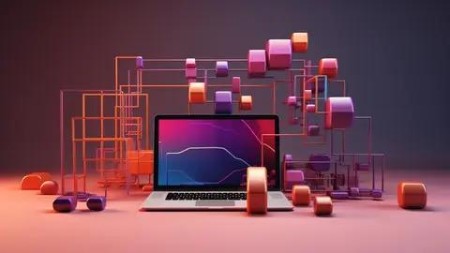
jаvascript Pro: Mastering Advanced Concepts And Techniques
Published 12/2023
MP4 | Video: h264, 1920x1080 | Audio: AAC, 44.1 KHz
Language: English | Size: 6.56 GB | Duration: 19h 0m
Level Up Your JS. Covers latest syntax, design patterns, functional programming, browser APIS, OOP, Canvas, and more!
What you'll learn
Explore Advanced jаvascript Patterns and Practices: proxy objects, observers, generators, and more
Apply Advanced Functional Programming Techniques: currying, composition, and more
Understand Scope, Closures, and Hoisting
Work with jаvascript APIs including Intersection Observers, Canvas, Web Sockets, and more
Master the trickiest parts of jаvascript
Learn the latest ES2021 & ES2022 features
Requirements
Basic jаvascript knowledge: familiarity with functions, loops, conditionals, etc.
Description
Transform your basic jаvascript knowledge into expert-level skills with this brand-new comprehensive course designed for those ready to take the next big leap in their programming career. If you've ever found yourself intimidated by jаvascript's more complex features or struggled to grasp its intricate concepts, this course is tailor-made for you. If you've taken a few Udemy courses on jаvascript and don't know where to go next, this course is for you! This course demystifies the 'scary' and tricky parts of jаvascript, guiding you through the intricate details and advanced aspects with ease. By the end of this journey, you'll not only understand these concepts but also skillfully apply them in real-world scenarios.Key Topics Covered:Object-Oriented Programming (OOP): SOLID design principles, prototypes, private class fields, etc.jаvascript Design Patterns: Proxy objects, module pattern, singleton pattern, observer pattern, mixin pattern, registry pattern, and others.Advanced jаvascript APIs: IndexedDB, Geolocation, Web Sockets, Notifications API, Canvas, getUserMedia, and more.'this' Keyword Mastery: Deep dive into 'this', call, apply, and bind methods.Asynchronous Programming: Master promises, async/await, asynchronous design patterns, and write your own promise objectsModern jаvascript Features: Optional chaining, nullish coalescing, logical assignment operators, and other ES2021 & ES2022 featuresTricky Parts of jаvascript: Tackle closures, float imprecision, BigInt, automatic semicolon insertion and a bunch more.Functional Programming Techniques: Recursion, currying, composition, partial application, and more.Whether you're a self-taught programmer, a computer science student, or a professional developer looking to sharpen your jаvascript skills, this course will elevate your coding abilities, preparing you to handle advanced web development challenges with confidence and expertise.
Overview
Section 1: Introduction
Lecture 1 Course Welcome & Introduction
Lecture 2 Curriculum Walkthrough
Lecture 3 Getting The Course Code
Lecture 4 My Developer Environment
Section 2: Object Oriented jаvascript
Lecture 5 Working With Plain Old jаvascript Objects
Lecture 6 Mixing Data & Functions With Objects
Lecture 7 Class Basics
Lecture 8 Constructors
Lecture 9 Practice Time: Bank Account
Lecture 10 Instance Methods
Lecture 11 Inheritance Basics
Lecture 12 The Super Keyword
Lecture 13 Static Properties
Lecture 14 Static Methods
Lecture 15 Use Cases For Static Methods
Lecture 16 Connect Four OO Exercise
Section 3: OOP: Newer Features in jаvascript
Lecture 17 Getters
Lecture 18 Setters
Lecture 19 Practice Time: Getters and Setters
Lecture 20 Public Fields
Lecture 21 Private Fields
Lecture 22 Private Methods
Lecture 23 ES2022 Static Initialization Blocks
Section 4: The Mysterious Keyword "This"
Lecture 24 Introducing This
Lecture 25 The Mystery of The Keyword This
Lecture 26 Global Objects and This
Lecture 27 The "Left Of The Dot" Rule
Lecture 28 This and Classes
Lecture 29 The Call Method
Lecture 30 The Apply Method
Lecture 31 The Bind Method
Lecture 32 Binding Arguments
Lecture 33 Bind With Event Listeners
Lecture 34 Bind With Timers
Lecture 35 Arrow Functions and This
Lecture 36 This Takeaways
Section 5: OOP Under The Hood: Prototypes, New, & More!
Lecture 37 OOP Under The Hood Intro
Lecture 38 The New Keyword
Lecture 39 Prototypes: Part 1
Lecture 40 Prototypes: Part 2
Lecture 41 Prototypes: Part 3
Lecture 42 The Prototype Chain
Lecture 43 Classes, Inheritance, & Prototypes
Lecture 44 __proto__ vs. prototype
Lecture 45 Useful Prototype Methods
Section 6: Asynchronous Code
Lecture 46 Callbacks: Our Good Friend
Lecture 47 Callback Hell & The Pyramid of Doom
Lecture 48 The Basics of Promises
Lecture 49 Using .then() and .catch()
Lecture 50 Promise Chaining To Flatten Code
Lecture 51 Error Handling With Promises
Lecture 52 Async/Await Basics
Lecture 53 More on Async/Await
Lecture 54 Error Handling With Async Functions
Lecture 55 Async Patterns: Parallel Async Operations
Lecture 56 Async Patterns: Sequential Async Operations
Lecture 57 Async Patterns: Promise.all()
Lecture 58 Async Patterns: Promise.allSettled()
Lecture 59 Async Patterns: Promise.race()
Lecture 60 Building Our Own Promise Objects
Lecture 61 Promisifying Node's fs.readFile()
Lecture 62 Async Num Cards Exercise
Section 7: Latest jаvascript Features
Lecture 63 Optional Chaining
Lecture 64 Nullish Coalescing
Lecture 65 Numeric Separators
Lecture 66 Array.prototype.at()
Lecture 67 String replaceAll()
Lecture 68 Logical OR Assignment ||=
Lecture 69 Logical AND Assignment &&=
Lecture 70 Nullish Coalescing Assignment ??=
Lecture 71 Promise.any()
Lecture 72 New OOP Features: Private Fields & Static Initialization Blocks
Section 8: The Tricky Parts of jаvascript
Lecture 73 Working With Float Imprecision
Lecture 74 BigInt() and Really Large Numbers
Lecture 75 isNan() vs. Number.isNaN()
Lecture 76 Post and Pre Increment: ++x vs. x++
Lecture 77 Automatic Semicolon Insertion
Lecture 78 jаvascript Generator Functions
Lecture 79 When Are Generators Useful?
Lecture 80 The Incredibly Versatile Array.from() Method
Section 9: The Tricky Parts: Scope & Closures
Lecture 81 Recaping Var & Scope
Lecture 82 Recaping Let, Const, & Scope
Lecture 83 The Scope Chain
Lecture 84 Static Scope
Lecture 85 Hoisting
Lecture 86 IIFEs
Lecture 87 Closures: The Basics
Lecture 88 Closures: Another Example
Lecture 89 Closures: Factory Functions
Lecture 90 Closures: Event Listeners
Lecture 91 Closures: Loops
Section 10: Timers: Debouncing, Throttling, & RequestAnimationFrame
Lecture 92 setTimeout
Lecture 93 setInterval
Lecture 94 clearInterval
Lecture 95 clearTimeout
Lecture 96 Debouncing
Lecture 97 Writing a Fancy Debounce Function
Lecture 98 Throttling
Lecture 99 Building a Fancy Throttle Function
Lecture 100 requestAnimationFrame Basics
Lecture 101 requestAnimationFrame With Timestamps
Lecture 102 Scroll To Top Animation With RequestAnimationFrame
Section 11: Functional Programming
Lecture 103 Introducing Functional Programming
Lecture 104 First Class Functions
Lecture 105 Writing Pure Functions
Lecture 106 Returning Functions
Lecture 107 Immutability
Lecture 108 Recursion
Lecture 109 Partial Application With Bind
Lecture 110 Writing a Partial Function
Lecture 111 Composition Basics
Lecture 112 A Simple Compose Function
Lecture 113 Writing a Fancier Compose Function
Lecture 114 Currying Basics
Lecture 115 More Advanced Currying
Lecture 116 Dice Game Intro
Lecture 117 Dice Game Simple FP
Lecture 118 Dice Game Going Overboard with FP
Lecture 119 Functional Programming Wrapup
Lecture 120 FP Stylizer Exercise
Section 12: Fetch API
Lecture 121 The Basics of Fetch
Lecture 122 Error Handling With Fetch
Lecture 123 Sending Request Headers With Fetch
Lecture 124 POST Requests With Fetch
Lecture 125 Uploading Files With Fetch
Lecture 126 JSON Placeholder Exercise
Section 13: Web Storage APIs
Lecture 127 LocalStorage Basics
Lecture 128 LocalStorage With Complex Objects
Lecture 129 What Should & Should Not Go In LocalStorage
Lecture 130 Creating a Darkmode Toggle With LocalStorage
Lecture 131 Localstorage Notes App Demo
Lecture 132 Syncing Tabs With The Storage Event
Lecture 133 Sessionstorage Basics
Lecture 134 Session Storage Form Demo
Lecture 135 IndexedDB: Enter At Your Own Risk!
Section 14: Browser APIs: Geolocation, Intersection Observers, and More!
Lecture 136 Using the Geolocation API
Lecture 137 The getUserMedia API
Lecture 138 Intersection Observers: Basics
Lecture 139 Intersection Observers: Thresholds
Lecture 140 Intersection Observers: Tracking Ad View Time
Lecture 141 Intersection Observers: Multiple Entries
Lecture 142 Intersection Observers: Lazy Loading Images
Section 15: Performance API & Web Audio
Lecture 143 Performance API Basics
Lecture 144 Measuring Resource Load Times With Performance API
Lecture 145 The Web Audio API Basics
Lecture 146 Web Audio Theremin Slider Demo
Lecture 147 Web Audio API Use Cases
Section 16: Canvas API
Lecture 148 Intro To The Canvas API
Lecture 149 Canvas Basics:
Lecture 150 Canvas Strokes and Lines
Lecture 151 Canvas Arcs and Paths
Lecture 152 Other Canvas Features
Lecture 153 Building A Bouncing Balls Toy Pt 1
Lecture 154 Building A Bouncing Balls Demo Pt 2
Lecture 155 Building A Bouncing Balls Demo Pt 3
Section 17: Web Sockets API
Lecture 156 Introducing Web Sockets
Lecture 157 Basics Of The Web Sockets API
Lecture 158 Web Socket Events
Lecture 159 Building A Chat App With Web Sockets: Pt 1
Lecture 160 Building A Chat App With Web Sockets: Pt 2
Lecture 161 Building A Chat App With Web Sockets: Pt 3
Section 18: Notifications API
Lecture 162 Sending Notifications
Lecture 163 Notification Events
Lecture 164 Notifications With Icons and Data
Lecture 165 Adding Notifications To Our Chat App: Pt. 1
Lecture 166 Adding Notifications To Our Chat App: Pt. 2
Section 19: SOLID OOP Principles
Lecture 167 Single Responsibility Principle
Lecture 168 Open/Closed Principle
Lecture 169 Liskov Substitution Principle
Lecture 170 Interface Segregation Principle
Lecture 171 Dependency Inversion Principle
Lecture 172 Law Of Demeter
Section 20: Design Patterns & Proxy Objects
Lecture 173 Module Pattern
Lecture 174 Singleton Pattern
Lecture 175 Observer Pattern
Lecture 176 Registry Pattern
Lecture 177 Mixin Pattern
Lecture 178 Proxy Pattern With Proxy Objects
Lecture 179 Proxying Function Calls
Lecture 180 Implementing Data Binding With Proxy Objects
Anyone looking to level up their jаvascript skills to a professional level
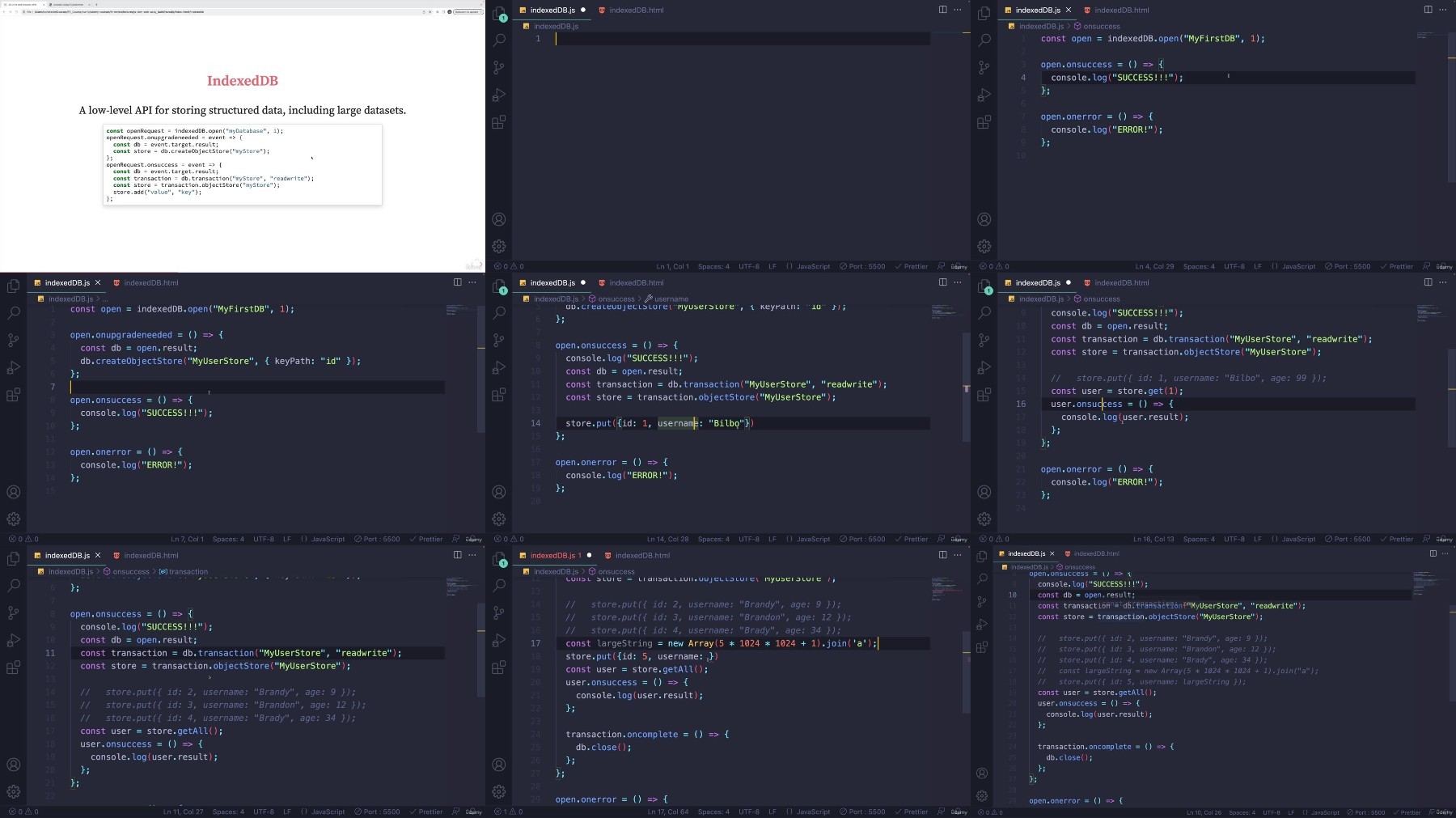
https://rapidgator.net/file/60f426cae25de55ee95e61727f8bfc59/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part1.rar
https://rapidgator.net/file/dd3ff1ac14989b28b8bc6c247e08a372/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part2.rar
https://rapidgator.net/file/2c953ad135a1d1ef78d47f855f821ee7/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part3.rar
https://filestore.me/0qutch48a02f/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part1.rar
https://filestore.me/8fy7wrbvqiy8/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part2.rar
https://filestore.me/0so2znurul0b/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part3.rar
What you'll learn
Explore Advanced jаvascript Patterns and Practices: proxy objects, observers, generators, and more
Apply Advanced Functional Programming Techniques: currying, composition, and more
Understand Scope, Closures, and Hoisting
Work with jаvascript APIs including Intersection Observers, Canvas, Web Sockets, and more
Master the trickiest parts of jаvascript
Learn the latest ES2021 & ES2022 features
Requirements
Basic jаvascript knowledge: familiarity with functions, loops, conditionals, etc.
Description
Transform your basic jаvascript knowledge into expert-level skills with this brand-new comprehensive course designed for those ready to take the next big leap in their programming career. If you've ever found yourself intimidated by jаvascript's more complex features or struggled to grasp its intricate concepts, this course is tailor-made for you. If you've taken a few Udemy courses on jаvascript and don't know where to go next, this course is for you! This course demystifies the 'scary' and tricky parts of jаvascript, guiding you through the intricate details and advanced aspects with ease. By the end of this journey, you'll not only understand these concepts but also skillfully apply them in real-world scenarios.Key Topics Covered:Object-Oriented Programming (OOP): SOLID design principles, prototypes, private class fields, etc.jаvascript Design Patterns: Proxy objects, module pattern, singleton pattern, observer pattern, mixin pattern, registry pattern, and others.Advanced jаvascript APIs: IndexedDB, Geolocation, Web Sockets, Notifications API, Canvas, getUserMedia, and more.'this' Keyword Mastery: Deep dive into 'this', call, apply, and bind methods.Asynchronous Programming: Master promises, async/await, asynchronous design patterns, and write your own promise objectsModern jаvascript Features: Optional chaining, nullish coalescing, logical assignment operators, and other ES2021 & ES2022 featuresTricky Parts of jаvascript: Tackle closures, float imprecision, BigInt, automatic semicolon insertion and a bunch more.Functional Programming Techniques: Recursion, currying, composition, partial application, and more.Whether you're a self-taught programmer, a computer science student, or a professional developer looking to sharpen your jаvascript skills, this course will elevate your coding abilities, preparing you to handle advanced web development challenges with confidence and expertise.
Overview
Section 1: Introduction
Lecture 1 Course Welcome & Introduction
Lecture 2 Curriculum Walkthrough
Lecture 3 Getting The Course Code
Lecture 4 My Developer Environment
Section 2: Object Oriented jаvascript
Lecture 5 Working With Plain Old jаvascript Objects
Lecture 6 Mixing Data & Functions With Objects
Lecture 7 Class Basics
Lecture 8 Constructors
Lecture 9 Practice Time: Bank Account
Lecture 10 Instance Methods
Lecture 11 Inheritance Basics
Lecture 12 The Super Keyword
Lecture 13 Static Properties
Lecture 14 Static Methods
Lecture 15 Use Cases For Static Methods
Lecture 16 Connect Four OO Exercise
Section 3: OOP: Newer Features in jаvascript
Lecture 17 Getters
Lecture 18 Setters
Lecture 19 Practice Time: Getters and Setters
Lecture 20 Public Fields
Lecture 21 Private Fields
Lecture 22 Private Methods
Lecture 23 ES2022 Static Initialization Blocks
Section 4: The Mysterious Keyword "This"
Lecture 24 Introducing This
Lecture 25 The Mystery of The Keyword This
Lecture 26 Global Objects and This
Lecture 27 The "Left Of The Dot" Rule
Lecture 28 This and Classes
Lecture 29 The Call Method
Lecture 30 The Apply Method
Lecture 31 The Bind Method
Lecture 32 Binding Arguments
Lecture 33 Bind With Event Listeners
Lecture 34 Bind With Timers
Lecture 35 Arrow Functions and This
Lecture 36 This Takeaways
Section 5: OOP Under The Hood: Prototypes, New, & More!
Lecture 37 OOP Under The Hood Intro
Lecture 38 The New Keyword
Lecture 39 Prototypes: Part 1
Lecture 40 Prototypes: Part 2
Lecture 41 Prototypes: Part 3
Lecture 42 The Prototype Chain
Lecture 43 Classes, Inheritance, & Prototypes
Lecture 44 __proto__ vs. prototype
Lecture 45 Useful Prototype Methods
Section 6: Asynchronous Code
Lecture 46 Callbacks: Our Good Friend
Lecture 47 Callback Hell & The Pyramid of Doom
Lecture 48 The Basics of Promises
Lecture 49 Using .then() and .catch()
Lecture 50 Promise Chaining To Flatten Code
Lecture 51 Error Handling With Promises
Lecture 52 Async/Await Basics
Lecture 53 More on Async/Await
Lecture 54 Error Handling With Async Functions
Lecture 55 Async Patterns: Parallel Async Operations
Lecture 56 Async Patterns: Sequential Async Operations
Lecture 57 Async Patterns: Promise.all()
Lecture 58 Async Patterns: Promise.allSettled()
Lecture 59 Async Patterns: Promise.race()
Lecture 60 Building Our Own Promise Objects
Lecture 61 Promisifying Node's fs.readFile()
Lecture 62 Async Num Cards Exercise
Section 7: Latest jаvascript Features
Lecture 63 Optional Chaining
Lecture 64 Nullish Coalescing
Lecture 65 Numeric Separators
Lecture 66 Array.prototype.at()
Lecture 67 String replaceAll()
Lecture 68 Logical OR Assignment ||=
Lecture 69 Logical AND Assignment &&=
Lecture 70 Nullish Coalescing Assignment ??=
Lecture 71 Promise.any()
Lecture 72 New OOP Features: Private Fields & Static Initialization Blocks
Section 8: The Tricky Parts of jаvascript
Lecture 73 Working With Float Imprecision
Lecture 74 BigInt() and Really Large Numbers
Lecture 75 isNan() vs. Number.isNaN()
Lecture 76 Post and Pre Increment: ++x vs. x++
Lecture 77 Automatic Semicolon Insertion
Lecture 78 jаvascript Generator Functions
Lecture 79 When Are Generators Useful?
Lecture 80 The Incredibly Versatile Array.from() Method
Section 9: The Tricky Parts: Scope & Closures
Lecture 81 Recaping Var & Scope
Lecture 82 Recaping Let, Const, & Scope
Lecture 83 The Scope Chain
Lecture 84 Static Scope
Lecture 85 Hoisting
Lecture 86 IIFEs
Lecture 87 Closures: The Basics
Lecture 88 Closures: Another Example
Lecture 89 Closures: Factory Functions
Lecture 90 Closures: Event Listeners
Lecture 91 Closures: Loops
Section 10: Timers: Debouncing, Throttling, & RequestAnimationFrame
Lecture 92 setTimeout
Lecture 93 setInterval
Lecture 94 clearInterval
Lecture 95 clearTimeout
Lecture 96 Debouncing
Lecture 97 Writing a Fancy Debounce Function
Lecture 98 Throttling
Lecture 99 Building a Fancy Throttle Function
Lecture 100 requestAnimationFrame Basics
Lecture 101 requestAnimationFrame With Timestamps
Lecture 102 Scroll To Top Animation With RequestAnimationFrame
Section 11: Functional Programming
Lecture 103 Introducing Functional Programming
Lecture 104 First Class Functions
Lecture 105 Writing Pure Functions
Lecture 106 Returning Functions
Lecture 107 Immutability
Lecture 108 Recursion
Lecture 109 Partial Application With Bind
Lecture 110 Writing a Partial Function
Lecture 111 Composition Basics
Lecture 112 A Simple Compose Function
Lecture 113 Writing a Fancier Compose Function
Lecture 114 Currying Basics
Lecture 115 More Advanced Currying
Lecture 116 Dice Game Intro
Lecture 117 Dice Game Simple FP
Lecture 118 Dice Game Going Overboard with FP
Lecture 119 Functional Programming Wrapup
Lecture 120 FP Stylizer Exercise
Section 12: Fetch API
Lecture 121 The Basics of Fetch
Lecture 122 Error Handling With Fetch
Lecture 123 Sending Request Headers With Fetch
Lecture 124 POST Requests With Fetch
Lecture 125 Uploading Files With Fetch
Lecture 126 JSON Placeholder Exercise
Section 13: Web Storage APIs
Lecture 127 LocalStorage Basics
Lecture 128 LocalStorage With Complex Objects
Lecture 129 What Should & Should Not Go In LocalStorage
Lecture 130 Creating a Darkmode Toggle With LocalStorage
Lecture 131 Localstorage Notes App Demo
Lecture 132 Syncing Tabs With The Storage Event
Lecture 133 Sessionstorage Basics
Lecture 134 Session Storage Form Demo
Lecture 135 IndexedDB: Enter At Your Own Risk!
Section 14: Browser APIs: Geolocation, Intersection Observers, and More!
Lecture 136 Using the Geolocation API
Lecture 137 The getUserMedia API
Lecture 138 Intersection Observers: Basics
Lecture 139 Intersection Observers: Thresholds
Lecture 140 Intersection Observers: Tracking Ad View Time
Lecture 141 Intersection Observers: Multiple Entries
Lecture 142 Intersection Observers: Lazy Loading Images
Section 15: Performance API & Web Audio
Lecture 143 Performance API Basics
Lecture 144 Measuring Resource Load Times With Performance API
Lecture 145 The Web Audio API Basics
Lecture 146 Web Audio Theremin Slider Demo
Lecture 147 Web Audio API Use Cases
Section 16: Canvas API
Lecture 148 Intro To The Canvas API
Lecture 149 Canvas Basics:
Lecture 150 Canvas Strokes and Lines
Lecture 151 Canvas Arcs and Paths
Lecture 152 Other Canvas Features
Lecture 153 Building A Bouncing Balls Toy Pt 1
Lecture 154 Building A Bouncing Balls Demo Pt 2
Lecture 155 Building A Bouncing Balls Demo Pt 3
Section 17: Web Sockets API
Lecture 156 Introducing Web Sockets
Lecture 157 Basics Of The Web Sockets API
Lecture 158 Web Socket Events
Lecture 159 Building A Chat App With Web Sockets: Pt 1
Lecture 160 Building A Chat App With Web Sockets: Pt 2
Lecture 161 Building A Chat App With Web Sockets: Pt 3
Section 18: Notifications API
Lecture 162 Sending Notifications
Lecture 163 Notification Events
Lecture 164 Notifications With Icons and Data
Lecture 165 Adding Notifications To Our Chat App: Pt. 1
Lecture 166 Adding Notifications To Our Chat App: Pt. 2
Section 19: SOLID OOP Principles
Lecture 167 Single Responsibility Principle
Lecture 168 Open/Closed Principle
Lecture 169 Liskov Substitution Principle
Lecture 170 Interface Segregation Principle
Lecture 171 Dependency Inversion Principle
Lecture 172 Law Of Demeter
Section 20: Design Patterns & Proxy Objects
Lecture 173 Module Pattern
Lecture 174 Singleton Pattern
Lecture 175 Observer Pattern
Lecture 176 Registry Pattern
Lecture 177 Mixin Pattern
Lecture 178 Proxy Pattern With Proxy Objects
Lecture 179 Proxying Function Calls
Lecture 180 Implementing Data Binding With Proxy Objects
Anyone looking to level up their jаvascript skills to a professional level
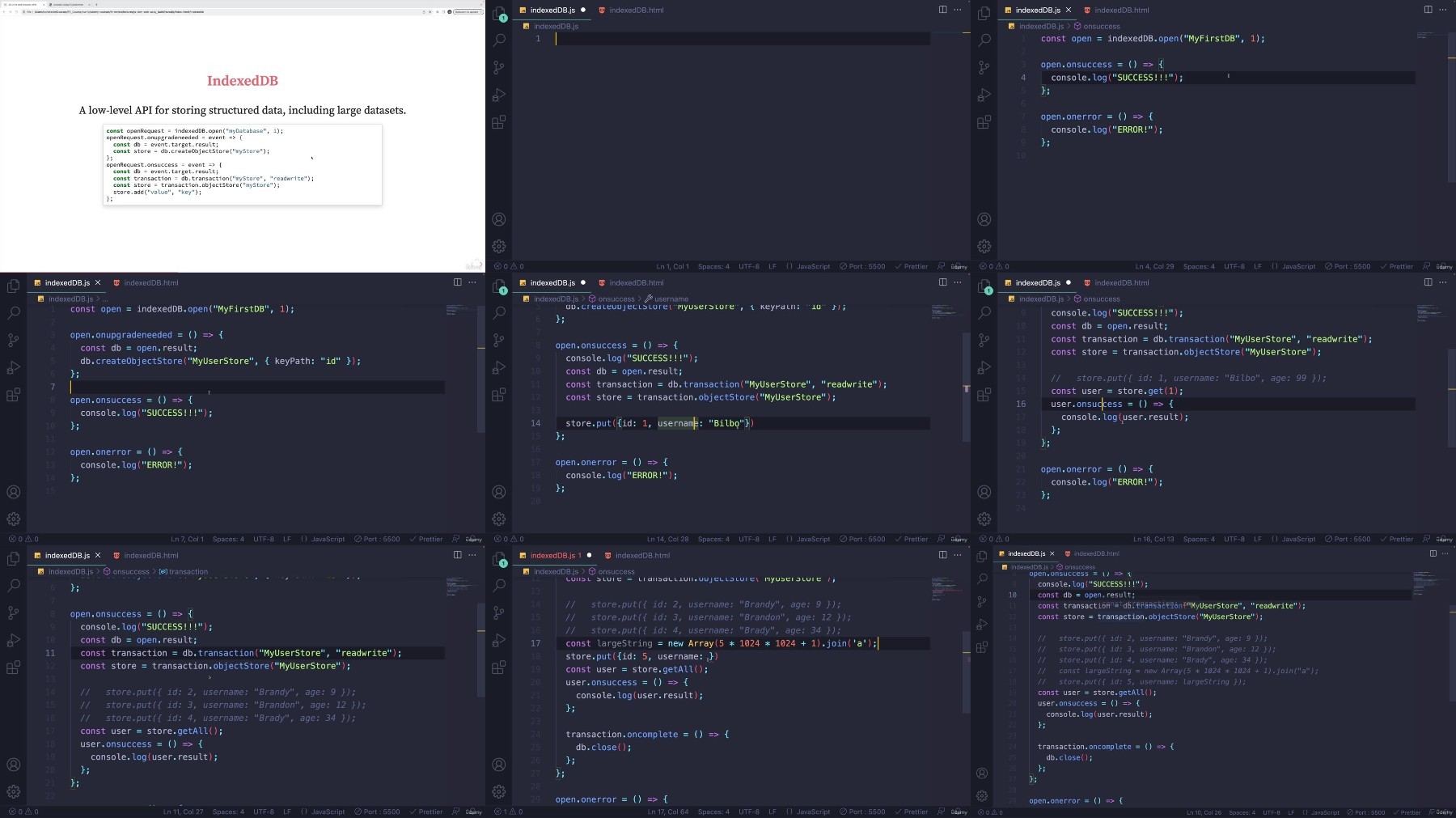
https://rapidgator.net/file/60f426cae25de55ee95e61727f8bfc59/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part1.rar
https://rapidgator.net/file/dd3ff1ac14989b28b8bc6c247e08a372/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part2.rar
https://rapidgator.net/file/2c953ad135a1d1ef78d47f855f821ee7/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part3.rar
https://filestore.me/0qutch48a02f/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part1.rar
https://filestore.me/8fy7wrbvqiy8/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part2.rar
https://filestore.me/0so2znurul0b/Udemy_jаvascript_Pro_Mastering_Advanced_Concepts_and_Techniques.part3.rar
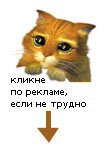